index.html
<html>
<head>
<meta http-equiv="content=type" content="text/html; charset=utf-8" />
</head>
<body>
<h1> 회원 관리 시스템</h1>
<a href="select.php"> (1) 회원 조회 (조회 후 수정/삭제 가능) </a><br /><br />
<a href="insert.php"> (2) 신규 회원 등록 </a><br /><br />
<form method="get" action="update.php">
(3) 회원 수정 - 회원 아이디 : <input type="text" name="userID" />
<input type="submit" value="수정" />
</form>
<form method="get" action="delete.php">
(4) 회원 삭제 - 회원 아이디 : <input type="text" name="userID" />
<input type="submit" value="삭제" />
</form>
</body>
</html>
select.php
<?php
header('Content-Type: text/html charset=utf-8');
con = mysqli_connect("localhost", "root", "1234", "sqlDB") or die("DB 접속 실패 !!"); sql = "SELECT * FROM userTBL";
ret = mysqli_query(con, sql); if (ret) {
echo mysqli_num_rows(ret), " 건이 조회됨.<br>" ; count = mysqli_num_rows(ret); } else { echo "userTBL 데이터 조회 실패!!<br>"; echo "실패 원인 : ", mysqli_error($con); echo "<br>"; exit(); } echo "<h1> 회원 조회 결과 </h1>"; echo "<TABLE border = 1>"; echo "<TR>"; echo "<TH>아이디</TH><TH>이름</TH><TH>출생년도</TH><TH>지역</TH><TH>국번</TH>"; echo "<TH>전화번호</TH><TH>키</TH><TH>가입일</TH><TH>수정</TH><TH>삭제</TH>"; echo "</TR>"; while (row = mysqli_fetch_array(ret)) { echo "<TR>"; echo "<TD>", $row['userID'], "</TD>"; echo "<TD>", $row['name'], "</TD>"; echo "<TD>", $row['birthYear'], "</TD>"; echo "<TD>", $row['addr'], "</TD>"; echo "<TD>", $row['mobile1'], "</TD>"; echo "<TD>", $row['mobile2'], "</TD>"; echo "<TD>", $row['height'], "</TD>"; echo "<TD>", $row['mDate'], "</TD>"; echo "<TD>", "<a href='update.php?userID=", $row['userID'], "'>수정</a></TD>"; echo "<TD>", "<a href='delete.php?userID=", $row['userID'], "'>삭제</a></TD>"; echo "</TR>"; } mysqli_close(con);
echo "</TABLE><br>";
echo "<a href='index.html'> <--초기 화면 </a>";
?>
insert.php
<?php
header('Content-Type: text/html charset=utf-8');
?>
<html>
<head>
<META http-equiv='content=type' content='text/html; charset=utf-8'>
</head>
<body>
<h1> 신규 회원 입력 </h1>
<form method='post' action='insert_result.php'>
아이디 : <input type='text' name='userID'> <br>
이름 : <input type='text' name='name'> <br>
출생년도 : <input type='text' name='birthYear'> <br>
지역 : <input type='text' name='addr'> <br>
휴대폰 국번 : <input type='text' name='mobile1'> <br>
휴대폰 전화번호 : <input type='text' name='mobile2'> <br>
신장 : <input type='text' name='height'> <br>
<br>
<input type='submit' value='회원 입력'>
</form>
</body>
</html>
insert_result.php
<?php
header('Content-Type: text/html charset=utf-8');
con = mysqli_connect("localhost", "root", "1234", "sqlDB") or die("DB 접속 실패 !!"); userID = _POST["userID"]; name = _POST["name"]; birthYear = _POST["birthYear"]; addr = _POST["addr"]; mobile1 = _POST["mobile1"]; mobile2 = _POST["mobile2"]; height = _POST["height"]; mDate = date("Y-m-d");
sql = "INSERT INTO userTBL VALUES('userID', 'name', 'birthYear', 'addr', 'mobile1', 'mobile2', 'height', 'mDate')"; ret = mysqli_query(con, sql);
echo "<h1> 신규 회원 입력 결과 </h1>";
if (ret) { echo "데이터가 성공적으로 입력됨.<br>"; } else { echo "데이터 입력 실패!!<br>"; echo "실패 원인 : ", mysqli_error($con); echo "<br>"; } mysqli_close(con);
echo "<a href='index.html'> <--초기 화면</a>";
?>
update.php
<?php
header('Content-Type: text/html charset=utf-8');
con = mysqli_connect("localhost", "root", "1234", "sqlDB") or die("DB 접속 실패 !!"); sql = "SELECT * FROM userTBL WHERE userID = '"._GET['userID']."'"; ret = mysqli_query(con, sql);
if (ret) { $count = mysqli_num_rows($ret); if ($count == 0) { echo $_GET['userID'], "아이디의 회원이 없음!!!<br>"; echo "<br> <a href='index.html'> <--초기 화면 </a>"; exit(); } } else { echo "데이터 조회 실패!!!<br>"; echo "실패 원인 : ", mysqli_error($con); echo "<br> <a href='index.html'> <--초기 화면 </a>"; exit(); } row = mysqli_fetch_array(ret); userID = row['userID']; name = row['name']; birthYear = row["birthYear"]; addr = row["addr"]; mobile1 = row["mobile1"]; mobile2 = row["mobile2"]; height = row["height"]; mDate = row["mDate"]; ?> <html> <head> <meta http-equiv="content=type" content="text/html; charset=utf-8" /> </head> <body> <h1> 회원 정보 수정 </h1> <form method='post' action='update_result.php'> 아이디 : <input type='text' name='userID' value=<?php echo userID ?> READONLY> <br>
이름 : <input type='text' name='name' value=<?php echo name ?>> <br> 출생년도 : <input type='text' name='birthYear' value=<?php echo birthYear ?>> <br>
지역 : <input type='text' name='addr' value=<?php echo addr ?>> <br> 휴대폰 국번 : <input type='text' name='mobile1' value=<?php echo mobile1 ?>> <br>
휴대폰 전화번호 : <input type='text' name='mobile2' value=<?php echo mobile2 ?>> <br> 신장 : <input type='text' name='height' value=<?php echo height ?>> <br>
회원가입일 : <input type='text' name='mDate' value=<?php echo $mDate ?> READONLY> <br>
<br>
<input type='submit' value='정보 수정'>
</form>
</body>
</html>
update_result.php
<?php
header('Content-Type: text/html charset=utf-8');
con = mysqli_connect("localhost", "root", "1234", "sqlDB") or die("DB 접속 실패 !!"); userID = _POST["userID"]; name = _POST["name"]; birthYear = _POST["birthYear"]; addr = _POST["addr"]; mobile1 = _POST["mobile1"]; mobile2 = _POST["mobile2"]; height = _POST["height"]; mDate = _POST["mDate"]; sql = "UPDATE userTBL SET name='".name."', birthYear='".birthYear."',
addr='".addr."', mobile1='".mobile1."', mobile2='".mobile2."', height='".height."', mDate='".mDate."' WHERE userID='".userID."'";
ret = mysqli_query(con, sql); echo "<h1> 회원 정보 수정 결과 </h1>"; if (ret) {
echo "데이터가 성공적으로 수정됨.";
}
else {
echo "데이터 수정 실패!!!<br>";
echo "실패 원인 : ", mysqli_error(con); echo "<br>"; } mysqli_close(con);
echo "<br> <a href='index.html'> <--초기 화면 </a>";
?>
delete.php
<?php
header('Content-Type: text/html charset=utf-8');
con = mysqli_connect("localhost", "root", "1234", "sqlDB") or die("DB 접속 실패 !!"); sql = "SELECT * FROM userTBL WHERE userID = '"._GET['userID']."'"; ret = mysqli_query(con, sql);
if (ret) { $count = mysqli_num_rows($ret); if ($count == 0) { echo $_GET['userID'], "아이디의 회원이 없음!!!<br>"; echo "<br> <a href='index.html'> <--초기 화면 </a>"; exit(); } } else { echo "데이터 조회 실패!!!<br>"; echo "실패 원인 : ", mysqli_error($con); echo "<br> <a href='index.html'> <--초기 화면 </a>"; exit(); } row = mysqli_fetch_array(ret); userID = row['userID']; name = row['name']; ?> <html> <head> <meta http-equiv="content=type" content="text/html; charset=utf-8" /> </head> <body> <h1> 회원 삭제 </h1> <form method='post' action='delete_result.php'> 아이디 : <input type='text' name='userID' value=<?php echo userID ?> READONLY> <br>
이름 : <input type='text' name='name' value=<?php echo $name ?>> <br>
<br>
위 회원을 삭제하겠습니까?
<input type='submit' value='회원 삭제'>
</form>
</body>
</html>
delete_result.php
<?php
header('Content-Type: text/html charset=utf-8');
con = mysqli_connect("localhost", "root", "1234", "sqlDB") or die("DB 접속 실패 !!"); userID = _POST["userID"]; sql = "DELETE FROM userTBL WHERE userID='".userID."'"; ret = mysqli_query(con, sql);
echo "<h1> 회원 삭제 결과 </h1>";
if (ret) { echo "회원이 성공적으로 삭제됨."; } else { echo "데이터 삭제 실패!!!<br>"; echo "실패 원인 : ", mysqli_error($con); echo "<br>"; } mysqli_close(con);
echo "<br> <a href='index.html'> <--초기 화면 </a>";
?>
결과 페이지
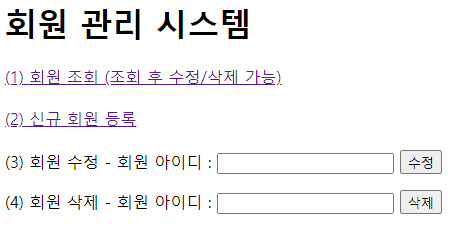
'🔐 [정보보안] 모의해킹 침해대응 전문가 취업캠프 > Web' 카테고리의 다른 글
[DB & PHP] PHP로 DB 접근 (0) | 2023.11.07 |
---|---|
[HTML & PHP] POST & GET 방식 (0) | 2023.11.06 |
[PHP] PHP 기본 문법 (0) | 2023.11.06 |
[HTML] HTML 기본 문법 (0) | 2023.11.06 |
[Database] 환경 변수 설정 (0) | 2023.11.02 |